이 자료는 로봇만들기 재능봉사단 활동에 사용될 자료입니다.
아두이노 핑퐁게임 만들기 키트를 구매하고, 포함된 교육자료를 인용하였습니다.
핑퐁게임 키트 구매 링크
아두이노 핑퐁게임 만들기는 컴퓨터와 내가 1:1 핑퐁 플레이를 하는 작품입니다.
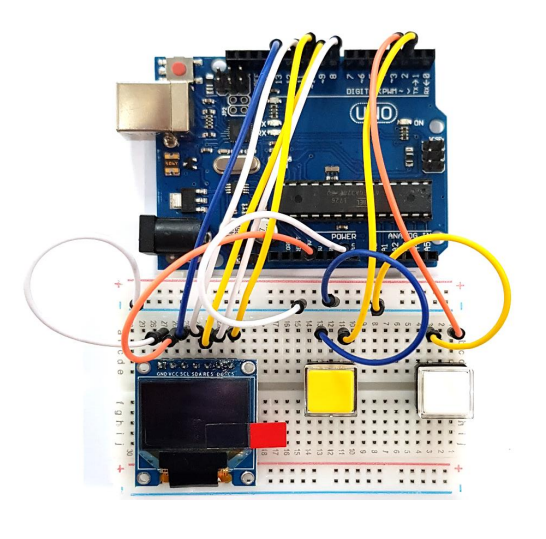
키트 안에 아래 부품이 들어있는지 먼저 확인해주세요.
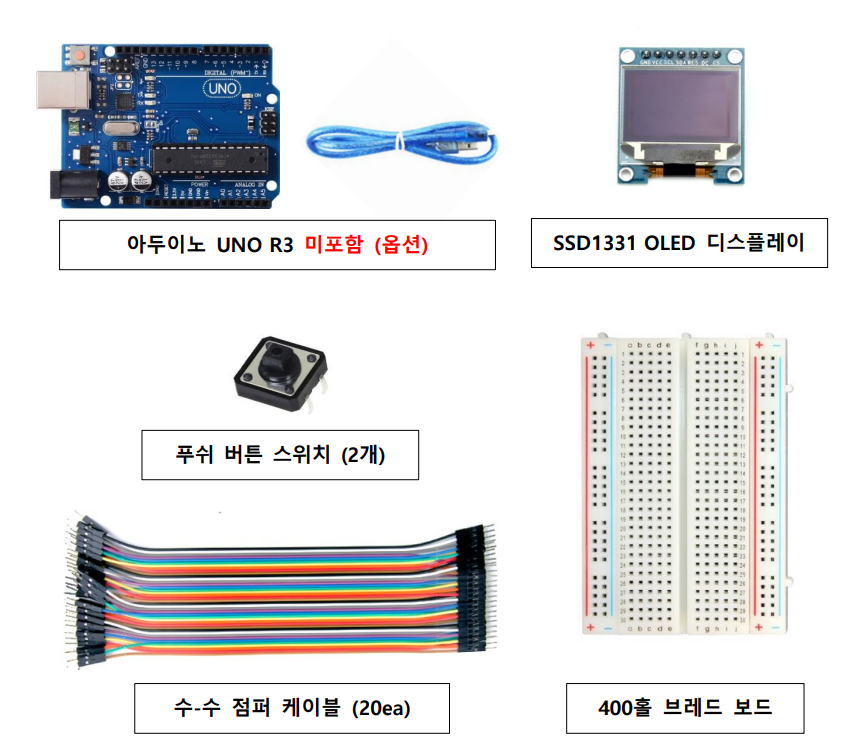
부품이 모두 제대로 들어있는 것을 확인했다면, 푸쉬버튼 스위치와 OLED 디스플레이에 대해서 알아봅시다.
먼저 푸쉬버튼 스위치부터 알아봅시다.
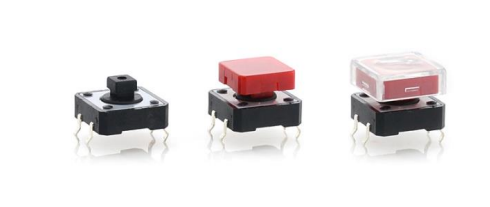
버튼을 누름으로써 접점을 개폐하는 스위치를 푸쉬버튼 스위치라고 하며 상단에 단추 모양의
버튼과 하단에 4 개의 단자로 구성되어 있습니다.
상단 버튼은 힘을 가하여 접점을 개폐하는 역할을 하며, 하단의 단자는 회로와
스위치를 연결하는 다리 역할을 합니다.
단자 연결은 마주보는 단자끼리 서로 연결되어 있어 한 쌍으로 구성됩니다.
아래 사진으로 예를 들면 기본상태에서는 서로 마주보고 있는 A 와 D 가 서로
연결되어 있고 B 와 C 가 서로 연결되어 있습니다.
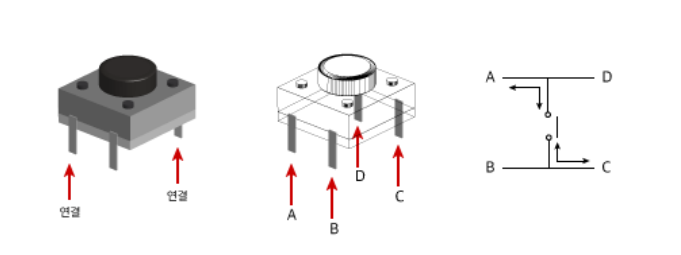
버튼을 누르지 않은 상태에서는 A, D 와 B, C 는 서로 떨어져 있지만 버튼을 누르게
되며 접점이 닿게 되어 A, D 와 B, C 가 서로 연결됩니다.
다음은 OLED 디스플레이입니다.
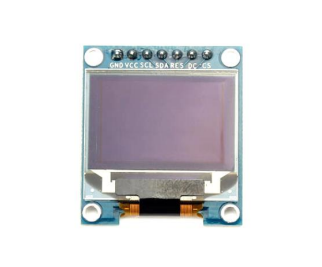
0.95 인치 RGB OLED 디스플레이입니다.
96×64 해상도를 가졌으며, 65536 컬러를 표현할 수 있는 RGB 디스플레이입니다.
LCD 드라이버는 SSD1331 을 탑재하고 있으며 3.3V/5V 에서 동작합니다.
푸쉬버튼 스위치가 제대로 동작하는지 테스트해봅시다.
아래와 같이 회로도를 연결해보세요.
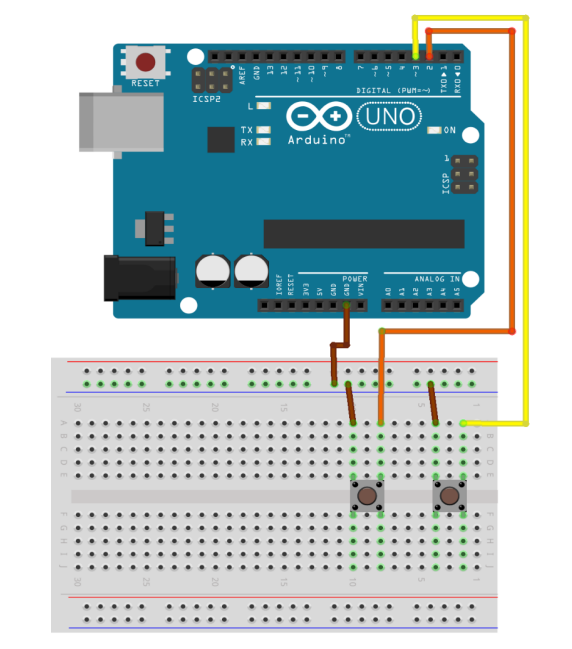
아래 코드를 아두이노에서 업로드합니다.
int Switch1 = 2; int Switch2 = 3; void setup() { pinMode(Switch1, INPUT_PULLUP); pinMode(Switch2, INPUT_PULLUP); Serial.begin(9600); } void loop() { int buttonState = digitalRead(2); int buttonstate = digitalRead(3); Serial.print(buttonState); Serial.println(buttonstate); delay(100); }
업로드 하고 스위치 버튼을 눌렀을때 아두이노 시리얼 모니터에 1이 나오는지 확인해보세요.
나오지 않는다면 회로를 잘못 연결했거나, 스위치가 고장났을 수 있습니다. 그게 아니라면 본인이 고장났을 수도 있으니 확인해보세요.
다음은 OLED 디스플레이가 제대로 작동하는지 확인해보겠습니다.
아래와 같이 회로를 연결해주세요.
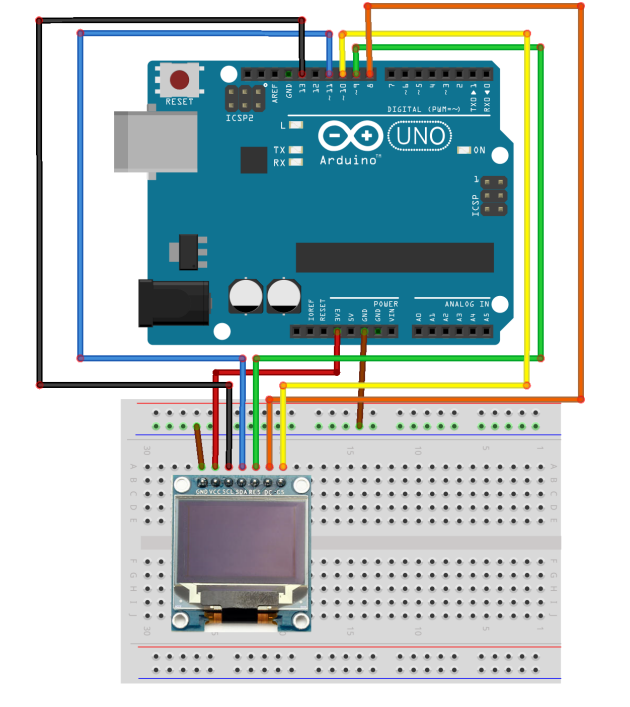
아래 코드를 아두이노에서 업로드 해줍니다.
/*************************************************** This is a example sketch demonstrating the graphics capabilities of the SSD1331 library for the 0.96" 16-bit Color OLED with SSD1331 driver chip Pick one up today in the adafruit shop! ------> http://www.adafruit.com/products/684 These displays use SPI to communicate, 4 or 5 pins are required to interface Adafruit invests time and resources providing this open source code, please support Adafruit and open-source hardware by purchasing products from Adafruit! Written by Limor Fried/Ladyada for Adafruit Industries. BSD license, all text above must be included in any redistribution ****************************************************/ #include <Adafruit_GFX.h> #include <Adafruit_SSD1331.h> #include <SPI.h> // You can use any (4 or) 5 pins #define sclk 13 #define mosi 11 #define cs 10 #define rst 9 #define dc 8 // Color definitions #define BLACK 0x0000 #define BLUE 0x001F #define RED 0xF800 #define GREEN 0x07E0 #define CYAN 0x07FF #define MAGENTA 0xF81F #define YELLOW 0xFFE0 #define WHITE 0xFFFF // Option 1: use any pins but a little slower //Adafruit_SSD1331 display = Adafruit_SSD1331(cs, dc, mosi, sclk, rst); // Option 2: must use the hardware SPI pins // (for UNO thats sclk = 13 and sid = 11) and pin 10 must be // an output. This is much faster - also required if you want // to use the microSD card (see the image drawing example) Adafruit_SSD1331 display = Adafruit_SSD1331(&SPI, cs, dc, rst); float p = 3.1415926; void setup(void) { Serial.begin(9600); Serial.print("hello!"); display.begin(); Serial.println("init"); uint16_t time = millis(); display.fillScreen(BLACK); time = millis() - time; Serial.println(time, DEC); delay(500); lcdTestPattern(); delay(1000); display.fillScreen(BLACK); display.setCursor(0,0); display.print("Lorem ipsum dolor sit amet, consectetur adipiscing elit. Curabitur adipiscing ante sed nibh tincidunt feugiat. Maecenas enim massa"); delay(1000); // tft print function! tftPrintTest(); delay(2000); //a single pixel display.drawPixel(display.width()/2, display.height()/2, GREEN); delay(500); // line draw test testlines(YELLOW); delay(500); // optimized lines testfastlines(RED, BLUE); delay(500); testdrawrects(GREEN); delay(1000); testfillrects(YELLOW, MAGENTA); delay(1000); display.fillScreen(BLACK); testfillcircles(10, BLUE); testdrawcircles(10, WHITE); delay(1000); testroundrects(); delay(500); testtriangles(); delay(500); Serial.println("done"); delay(1000); } void loop() { } void testlines(uint16_t color) { display.fillScreen(BLACK); for (int16_t x=0; x < display.width()-1; x+=6) { display.drawLine(0, 0, x, display.height()-1, color); } for (int16_t y=0; y < display.height()-1; y+=6) { display.drawLine(0, 0, display.width()-1, y, color); } display.fillScreen(BLACK); for (int16_t x=0; x < display.width()-1; x+=6) { display.drawLine(display.width()-1, 0, x, display.height()-1, color); } for (int16_t y=0; y < display.height()-1; y+=6) { display.drawLine(display.width()-1, 0, 0, y, color); } // To avoid ESP8266 watchdog timer resets when not using the hardware SPI pins delay(0); display.fillScreen(BLACK); for (int16_t x=0; x < display.width()-1; x+=6) { display.drawLine(0, display.height()-1, x, 0, color); } for (int16_t y=0; y < display.height()-1; y+=6) { display.drawLine(0, display.height()-1, display.width()-1, y, color); } display.fillScreen(BLACK); for (int16_t x=0; x < display.width()-1; x+=6) { display.drawLine(display.width()-1, display.height()-1, x, 0, color); } for (int16_t y=0; y < display.height()-1; y+=6) { display.drawLine(display.width()-1, display.height()-1, 0, y, color); } } void testdrawtext(char *text, uint16_t color) { display.setTextSize(1); display.setTextColor(WHITE); display.setCursor(0,0); for (uint8_t i=0; i < 168; i++) { if (i == '\n') continue; display.write(i); if ((i > 0) && (i % 21 == 0)) display.println(); } } void testfastlines(uint16_t color1, uint16_t color2) { display.fillScreen(BLACK); for (int16_t y=0; y < display.height()-1; y+=5) { display.drawFastHLine(0, y, display.width()-1, color1); } for (int16_t x=0; x < display.width()-1; x+=5) { display.drawFastVLine(x, 0, display.height()-1, color2); } } void testdrawrects(uint16_t color) { display.fillScreen(BLACK); for (int16_t x=0; x < display.height()-1; x+=6) { display.drawRect((display.width()-1)/2 -x/2, (display.height()-1)/2 -x/2 , x, x, color); } } void testfillrects(uint16_t color1, uint16_t color2) { display.fillScreen(BLACK); for (int16_t x=display.height()-1; x > 6; x-=6) { display.fillRect((display.width()-1)/2 -x/2, (display.height()-1)/2 -x/2 , x, x, color1); display.drawRect((display.width()-1)/2 -x/2, (display.height()-1)/2 -x/2 , x, x, color2); } } void testfillcircles(uint8_t radius, uint16_t color) { for (uint8_t x=radius; x < display.width()-1; x+=radius*2) { for (uint8_t y=radius; y < display.height()-1; y+=radius*2) { display.fillCircle(x, y, radius, color); } } } void testdrawcircles(uint8_t radius, uint16_t color) { for (int16_t x=0; x < display.width()-1+radius; x+=radius*2) { for (int16_t y=0; y < display.height()-1+radius; y+=radius*2) { display.drawCircle(x, y, radius, color); } } } void testtriangles() { display.fillScreen(BLACK); int color = 0xF800; int t; int w = display.width()/2; int x = display.height(); int y = 0; int z = display.width(); for (t = 0 ; t <= 15; t+=1) { display.drawTriangle(w, y, y, x, z, x, color); x-=4; y+=4; z-=4; color+=100; } } void testroundrects() { display.fillScreen(BLACK); int color = 100; int i; int t; for(t = 0 ; t <= 4; t+=1) { int x = 0; int y = 0; int w = display.width(); int h = display.height(); for(i = 0 ; i <= 8; i+=1) { display.drawRoundRect(x, y, w, h, 5, color); x+=2; y+=3; w-=4; h-=6; color+=1100; } color+=100; } } void tftPrintTest() { display.fillScreen(BLACK); display.setCursor(0, 5); display.setTextColor(RED); display.setTextSize(1); display.println("Hello World!"); display.setTextColor(YELLOW, GREEN); display.setTextSize(2); display.print("Hello Wo"); display.setTextColor(BLUE); display.setTextSize(3); display.print(1234.567); delay(1500); display.setCursor(0, 5); display.fillScreen(BLACK); display.setTextColor(WHITE); display.setTextSize(0); display.println("Hello World!"); display.setTextSize(1); display.setTextColor(GREEN); display.print(p, 5); display.println(" Want pi?"); display.print(8675309, HEX); // print 8,675,309 out in HEX! display.print(" Print HEX"); display.setTextColor(WHITE); display.println("Sketch has been"); display.println("running for: "); display.setTextColor(MAGENTA); display.print(millis() / 1000); display.setTextColor(WHITE); display.print(" seconds."); } void mediabuttons() { // play display.fillScreen(BLACK); display.fillRoundRect(25, 10, 78, 60, 8, WHITE); display.fillTriangle(42, 20, 42, 60, 90, 40, RED); delay(500); // pause display.fillRoundRect(25, 90, 78, 60, 8, WHITE); display.fillRoundRect(39, 98, 20, 45, 5, GREEN); display.fillRoundRect(69, 98, 20, 45, 5, GREEN); delay(500); // play color display.fillTriangle(42, 20, 42, 60, 90, 40, BLUE); delay(50); // pause color display.fillRoundRect(39, 98, 20, 45, 5, RED); display.fillRoundRect(69, 98, 20, 45, 5, RED); // play color display.fillTriangle(42, 20, 42, 60, 90, 40, GREEN); } /**************************************************************************/ /*! @brief Renders a simple test pattern on the LCD */ /**************************************************************************/ void lcdTestPattern(void) { uint8_t w,h; display.setAddrWindow(0, 0, 96, 64); for (h = 0; h < 64; h++) { for (w = 0; w < 96; w++) { if (w > 83) { display.writePixel(w, h, WHITE); } else if (w > 71) { display.writePixel(w, h, BLUE); } else if (w > 59) { display.writePixel(w, h, GREEN); } else if (w > 47) { display.writePixel(w, h, CYAN); } else if (w > 35) { display.writePixel(w, h, RED); } else if (w > 23) { display.writePixel(w, h, MAGENTA); } else if (w > 11) { display.writePixel(w, h, YELLOW); } else { display.writePixel(w, h, BLACK); } } } display.endWrite(); }
그런데 여기서 컴파일 하기 전에 라이브러리 두개를 포함시켜주어야 하는데요, 라이브러리 매니저에 들어가서 Adafruit GFX 라이브러리를 설치하고, Adafruit SSD1331 라이브러리를 차례로 설치해줍니다.
그래픽이 제대로 나오는지 확인해보세요.
두 부품이 모두 다 정상이라면, 이제 핑퐁 게임을 완성할 차례입니다.
아래와 같이 최종 핑퐁게임의 회로도를 보고 연결이 잘 되었는지 확인해주세요.
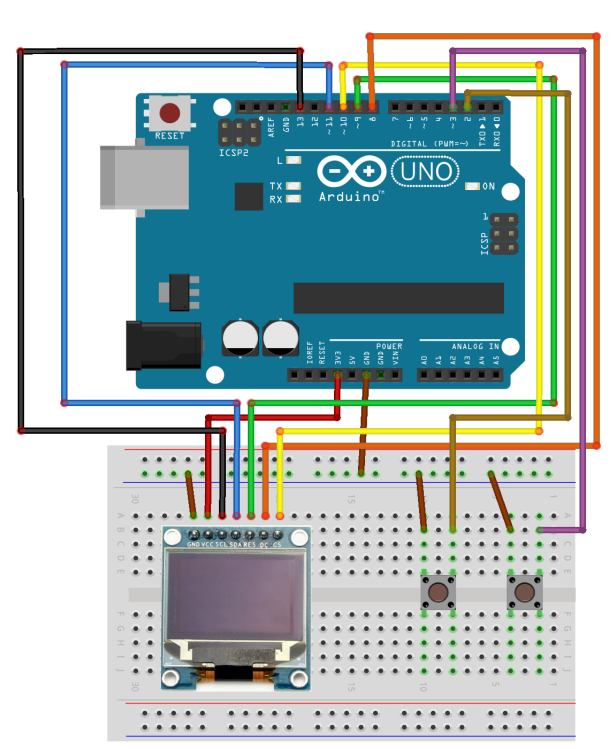
아래 코드를 업로드합니다.
#include <SPI.h> #include <Wire.h> #include <Adafruit_GFX.h> #include <Adafruit_SSD1331.h> #define UP_BUTTON 2 #define DOWN_BUTTON 3 // Color definitions #define BLACK 0x0000 #define BLUE 0x001F #define RED 0xF800 #define GREEN 0x07E0 #define CYAN 0x07FF #define MAGENTA 0xF81F #define YELLOW 0xFFE0 #define WHITE 0xFFFF const unsigned long PADDLE_RATE = 33; const unsigned long BALL_RATE = 20; const uint8_t PADDLE_HEIGHT = 24; int MAX_SCORE = 8; int CPU_SCORE = 0; int PLAYER_SCORE = 0; // You can use any (4 or) 5 pins #define sclk 13 #define mosi 11 #define cs 10 #define rst 9 #define dc 8 // MOSI is Data pin on display breakout Adafruit_SSD1331 display = Adafruit_SSD1331(cs, dc, rst); void drawCourt(); uint8_t ball_x = 64, ball_y = 32; uint8_t ball_dir_x = 1, ball_dir_y = 1; boolean gameIsRunning = true; boolean resetBall = false; static const unsigned char pong []PROGMEM = { 0xff,0xe0,0x0,0x3f,0x80,0x7,0xe0,0x7,0xc0,0x3,0xfc,0x0, 0xff,0xf8,0x1,0xff,0xe0,0x7,0xf0,0x7,0xc0,0x1f,0xff,0x0, 0xff,0xfc,0x3,0xff,0xf0,0x7,0xf0,0x7,0xc0,0x3f,0xff,0x0, 0xff,0xfe,0x7,0xff,0xf8,0x7,0xf8,0x7,0xc0,0xff,0xff,0x0, 0xf8,0x7f,0xf,0xff,0xfc,0x7,0xfc,0x7,0xc0,0xff,0xff,0x0, 0xf8,0x3f,0xf,0xe0,0xfe,0x7,0xfc,0x7,0xc1,0xfc,0x7,0x0, 0xf8,0x1f,0x1f,0x80,0x7e,0x7,0xfe,0x7,0xc3,0xf8,0x1,0x0, 0xf8,0x1f,0x1f,0x0,0x3e,0x7,0xfe,0x7,0xc3,0xf0,0x0,0x0, 0xf8,0x1f,0x3f,0x0,0x3f,0x7,0xdf,0x7,0xc7,0xe0,0x0,0x0, 0xf8,0x1f,0x3e,0x0,0x1f,0x7,0xdf,0x87,0xc7,0xc0,0x0,0x0, 0xf8,0x3f,0x3e,0x0,0x1f,0x7,0xcf,0x87,0xc7,0xc1,0xff,0x80, 0xf8,0x7e,0x3e,0x0,0x1f,0x7,0xc7,0xc7,0xc7,0xc1,0xff,0x80, 0xff,0xfe,0x3e,0x0,0x1f,0x7,0xc7,0xe7,0xc7,0xc1,0xff,0x80, 0xff,0xfc,0x3e,0x0,0x1f,0x7,0xc3,0xe7,0xc7,0xc1,0xff,0x80, 0xff,0xf8,0x3e,0x0,0x1f,0x7,0xc1,0xf7,0xc7,0xc0,0xf,0x80, 0xff,0xe0,0x3f,0x0,0x3f,0x7,0xc1,0xf7,0xc7,0xe0,0xf,0x80, 0xf8,0x0,0x1f,0x0,0x3e,0x7,0xc0,0xff,0xc3,0xe0,0xf,0x80, 0xf8,0x0,0x1f,0x80,0x7e,0x7,0xc0,0x7f,0xc3,0xf0,0xf,0x80, 0xf8,0x0,0x1f,0xc0,0xfc,0x7,0xc0,0x7f,0xc3,0xfc,0xf,0x80, 0xf8,0x0,0xf,0xff,0xfc,0x7,0xc0,0x3f,0xc1,0xff,0xff,0x80, 0xf8,0x0,0x7,0xff,0xf8,0x7,0xc0,0x3f,0xc0,0xff,0xff,0x80, 0xf8,0x0,0x3,0xff,0xf0,0x7,0xc0,0x1f,0xc0,0x7f,0xff,0x80, 0xf8,0x0,0x1,0xff,0xe0,0x7,0xc0,0xf,0xc0,0x3f,0xff,0x0, 0xf8,0x0,0x0,0x7f,0x0,0x7,0xc0,0xf,0xc0,0x7,0xf8,0x0 }; static const unsigned char game []PROGMEM ={ 0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x80, 0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x1,0xff,0x80, 0x0,0x0,0x0,0x0,0x0,0x0,0x0,0xc3,0xff,0x80, 0x0,0x0,0x0,0x0,0x0,0x0,0xf,0xc3,0xff,0x80, 0x0,0x0,0x0,0x0,0x3,0xe0,0xf,0xc3,0xe0,0x0, 0x0,0x0,0x0,0x0,0x7,0xf0,0x1f,0xc1,0xe0,0x0, 0x0,0x0,0x0,0xf8,0x7,0xf0,0x1f,0xc1,0xe0,0x0, 0x0,0xfc,0x1,0xfc,0x7,0xf8,0x1f,0xc1,0xe0,0x0, 0x7,0xfc,0x1,0xfc,0x3,0xf8,0x1f,0xe1,0xff,0x80, 0x1f,0xfc,0x1,0xde,0x3,0xbc,0x3d,0xe1,0xff,0x80, 0x3f,0xfe,0x1,0xde,0x3,0xbc,0x39,0xe1,0xff,0x80, 0x7e,0x0,0x3,0xdf,0x3,0xde,0x39,0xe1,0xfc,0x0, 0x7c,0x0,0x3,0xcf,0x3,0xde,0x39,0xe1,0xe0,0x0, 0xf8,0x0,0x3,0xcf,0x3,0xcf,0x39,0xe1,0xf0,0x0, 0xf8,0x0,0x3,0x87,0x83,0xcf,0x79,0xe0,0xf0,0x0, 0xf0,0x7f,0x7,0x87,0x83,0xc7,0xf1,0xe0,0xf0,0xe0, 0xf0,0xff,0x7,0x83,0xc3,0xc7,0xf1,0xe0,0xff,0xe0, 0xf0,0xff,0x7,0xff,0xc1,0xc3,0xf1,0xf0,0xff,0xe0, 0xf0,0xff,0x7,0xff,0xe1,0xc3,0xf0,0xf0,0xff,0xe0, 0xf8,0xf,0xf,0xff,0xe1,0xc1,0xe0,0xf0,0xe0,0x0, 0xf8,0xf,0x8f,0x1,0xf1,0xe1,0xe0,0xf0,0x0,0x0, 0x7c,0xf,0x8f,0x0,0xf1,0xe1,0xe0,0x0,0x0,0x0, 0x7f,0x1f,0x8f,0x0,0xf9,0xc0,0x0,0x0,0x0,0x0, 0x3f,0xff,0x9f,0x0,0x0,0x0,0x0,0x0,0x0,0x0, 0x1f,0xff,0x1f,0x0,0x0,0x0,0x0,0x0,0x0,0x0, 0x7,0xfc,0x0,0x0,0x0,0x0,0x0,0x0,0x0,0x0 }; unsigned long ball_update; unsigned long paddle_update; const uint8_t CPU_X = 12; uint8_t cpu_y = 16; const uint8_t PLAYER_X = 85; uint8_t player_y = 16; void setup() { pinMode(UP_BUTTON, INPUT_PULLUP); pinMode(DOWN_BUTTON, INPUT_PULLUP); display.begin(); display.fillScreen(BLACK); display.drawBitmap(3,0,pong,89,24,GREEN); display.drawBitmap(10,30,game,75,26,RED); while(digitalRead(UP_BUTTON) == HIGH && digitalRead(DOWN_BUTTON) == HIGH) { delay(100); } unsigned long start = millis(); display.fillScreen(BLACK); drawCourt(); while(millis() - start < 2000); ball_update = millis(); paddle_update = ball_update; ball_x = random(25,65); ball_y = random(3,63); } void loop() { unsigned long time = millis(); static bool up_state = false; static bool down_state = false; up_state |= (digitalRead(UP_BUTTON) == LOW); down_state |= (digitalRead(DOWN_BUTTON) == LOW); if(resetBall) { ball_x = random(25,70); ball_y = random(3,63); do { ball_dir_x = random(-1,2); }while(ball_dir_x==0); do { ball_dir_y = random(-1,2); }while(ball_dir_y==0); resetBall=false; } if(time > ball_update && gameIsRunning) { uint8_t new_x = ball_x + ball_dir_x; uint8_t new_y = ball_y + ball_dir_y; // Check if we hit the vertical walls if(new_x == 0) //Player Gets a Point { PLAYER_SCORE++; if(PLAYER_SCORE==MAX_SCORE) { gameOver(); }else { showScore(); } } // Check if we hit the vertical walls if(new_x == 95) //CPU Gets a Point { CPU_SCORE++; if(CPU_SCORE==MAX_SCORE) { gameOver(); }else { showScore(); } } // Check if we hit the horizontal walls. if(new_y == 0 || new_y == 63) { ball_dir_y = -ball_dir_y; new_y += ball_dir_y + ball_dir_y; } // Check if we hit the CPU paddle if(new_x == CPU_X && new_y >= cpu_y && new_y <= cpu_y + PADDLE_HEIGHT) { ball_dir_x = -ball_dir_x; new_x += ball_dir_x + ball_dir_x; } // Check if we hit the player paddle if(new_x == PLAYER_X && new_y >= player_y && new_y <= player_y + PADDLE_HEIGHT) { ball_dir_x = -ball_dir_x; new_x += ball_dir_x + ball_dir_x; } display.drawPixel(ball_x, ball_y, BLACK); display.drawPixel(new_x, new_y, WHITE); ball_x = new_x; ball_y = new_y; ball_update += BALL_RATE; } if(time > paddle_update && gameIsRunning) { paddle_update += PADDLE_RATE; // CPU paddle display.drawFastVLine(CPU_X, cpu_y,PADDLE_HEIGHT, BLACK); const uint8_t half_paddle = PADDLE_HEIGHT >> 1; if(cpu_y + half_paddle > ball_y) { cpu_y -= 1; } if(cpu_y + half_paddle < ball_y) { cpu_y += 1; } if(cpu_y < 1) cpu_y = 1; if(cpu_y + PADDLE_HEIGHT > 63) cpu_y = 63 - PADDLE_HEIGHT; display.drawFastVLine(CPU_X, cpu_y, PADDLE_HEIGHT, RED); // Player paddle display.drawFastVLine(PLAYER_X, player_y,PADDLE_HEIGHT, BLACK); if(up_state) { player_y -= 1; } if(down_state) { player_y += 1; } up_state = down_state = false; if(player_y < 1) player_y = 1; if(player_y + PADDLE_HEIGHT > 63) player_y = 63 - PADDLE_HEIGHT; display.drawFastVLine(PLAYER_X, player_y, PADDLE_HEIGHT, GREEN); } } void drawCourt() { display.drawRect(0, 0, 96, 64, WHITE); } void gameOver() { gameIsRunning = false; display.fillScreen(BLACK); drawCourt(); if(PLAYER_SCORE>CPU_SCORE) { display.setCursor(5,4); display.setTextColor(WHITE); display.setTextSize(2); display.print("You Won"); }else { display.setCursor(5,4); display.setTextColor(WHITE); display.setTextSize(2); display.print("CPU WON"); } display.setCursor(20,30); display.setTextColor(RED); display.setTextSize(3); display.print(String(CPU_SCORE)); display.setCursor(60,30); display.setTextColor(GREEN); display.setTextSize(3); display.print(String(PLAYER_SCORE)); delay(2000); while(digitalRead(UP_BUTTON) == HIGH && digitalRead(DOWN_BUTTON) == HIGH) { delay(100); } gameIsRunning = true; CPU_SCORE = PLAYER_SCORE = 0; unsigned long start = millis(); display.fillScreen(BLACK); drawCourt(); while(millis() - start < 2000); ball_update = millis(); paddle_update = ball_update; gameIsRunning = true; resetBall=true; } void showScore() { gameIsRunning = false; display.fillScreen(BLACK); drawCourt(); display.setCursor(15,4); display.setTextColor(WHITE); display.setTextSize(2); display.print("Score"); display.setCursor(20,30); display.setTextColor(RED); display.setTextSize(3); display.print(String(CPU_SCORE)); display.setCursor(60,30); display.setTextColor(GREEN); display.setTextSize(3); display.print(String(PLAYER_SCORE)); delay(2000); unsigned long start = millis(); display.fillScreen(BLACK); drawCourt(); while(millis() - start < 2000); ball_update = millis(); paddle_update = ball_update; gameIsRunning = true; resetBall=true; }
핑퐁게임은 왼쪽 버튼을 누르면 바가 올라가고 오른쪽 버튼을 누르면 바가 내려갑니다.
컴퓨터와 상대로 득점할 수 있도록 해보세요.